The most asked about question that I received at Interactions '14 was how to do REST in handlers. Adding REST toolsteps is not currently on the roadmap, so we have to make due with the tools we are given.
Making an Outbound Request
REST is not much more than plain HTTP, so to understand what needs to happen in our handlers lets start with a high level overview of HTTP. A HTTP request is a TCP data stream with the following format:
<request line>
<request headerrs>
<empty line>
[<message body>]
So if we look at an example HTTP message in Fiddler, it will look like this:
GET http://localhost:8018/icws/connection/features HTTP/1.1
Host: localhost:8018
Connection: keep-alive
Accept-Language: en-us
User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_9_2) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.153 Safari/537.36
Accept: */*
DNT: 1
Accept-Encoding: gzip,deflate,sdch
In this case, it is a GET request so there isn't a message body. If we were doing a POST or PUT there would be a blank line followed by the body content. Now that we know the formatting basics, we can translate that to a handler. Interaction Designer gives us five Tcp toolsteps in the File I/O category for us to work with.
Tcp Connect - Opens a TCP socket to a remote machine.
Tcp Read String - Reads a string from the TCP socket.
Tcp Write String - Writes a string from the TCP socket.
Tcp Close - Closes the TCP socket.
Tcp Listen - We'll cover this one in a little bit.
With these tools, we can create a data string following the HTTP standard, open a TCP socket, write our string to the socket, read the response and then close the socket.
Always make sure you are closing your socket. Example handlers be found in the GitHub repository
https://github.com/InteractiveIntelligence/RestThroughHandlers. An example of making an outbound request can be found in the handler RestMakeRequest. This handler takes an already opened socket, url, optional body, and lists for headers and will then construct the HTTP message and send it along the TCP socket. After we have send our data, we need to read it's response. The response is formatted similar to the request and the handler RestRead will take care of reading the data. There is a loop while reading the TCP data.
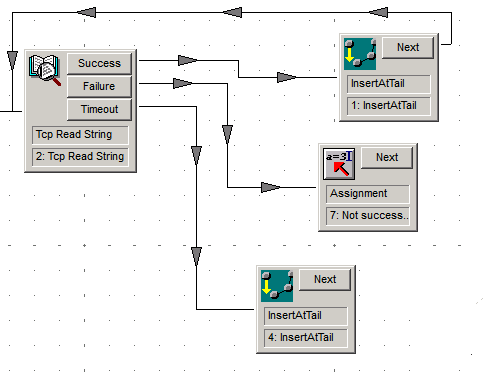
The Tcp Read String toolstep will read one line at a time and then the handler puts each line in a list. The handler assumes that it has reached the end of the data when the Tcp Read String toolstep times out. A better solution would be to look at the Content-Length header and then make sure that the data is that lenght, but for this example we can stick with the timeout. The rest of the RestRead handler parses out the header and body information.
Stringing these two helper handlers together, we end up with the RestTest handler. This handler will make a call to get the ICWS features supported on a CIC server.

The first step is to open our TCP connection, here Morbo is the name of the server and 8018 is the port that ICWS uses.
Next, we can call the RestMakeRequest subroutine. Note the method and the URL.
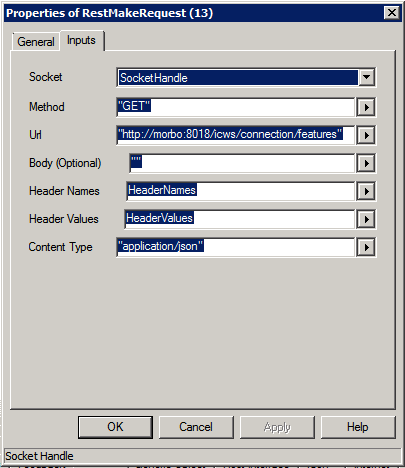
Now that the request has been made, we can use the RestRead subroutine to get the response and then close the socket with the Tcp Close toolstep. At the end of the handler, the ResponseBody variable should look something like this.
{"featureInfoList":[{"featureId":"activations","version":1},{"featureId":"statistics","version":1},{"featureId":"configuration","version":1},{"featureId":"connection","version":1},{"featureId":"directories","version":1},{"featureId":"interactions","version":1},{"featureId":"licenses","version":1},{"featureId":"messaging","version":1},{"featureId":"queues","version":1},{"featureId":"session","version":1},{"featureId":"status","version":1},{"featureId":"wfm","version":1}]}
There are two handlers included in this example which can help out with parsing and creating JSON. They are JSONToList which will take a JSON object and convert it into a list of names and a list of values, then ListToJSON which will create a JSON object given two lists. Both these helper handlers are for very simple JSON conversion and can't handle the response from our ICWS call so you may need to modify it to fit your needs.
Hosting A Web Service
Handlers will also let us set up a web listener and let us use the same concepts to listen to inbound REST requests. To start the listener, we need to call the Tcp Listen toolstep, this can be done either in a Custom Notification or System Initialization handler and the Tcp Listen toolstep just specifies a port to listen on.
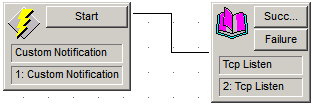
Whenever a TCP connection is established to one of the ports opened by the Tcp Listen toolstep, handlers with the TCP/IP Connection Accepted initiator will be called. The RestReceiver handler is an example of a listener handler. To receive HTTP messages the flow works opposite from sending the messages, we need to listen for data on the TCP socket and then send a response on the socket. In the RestReceiver handler, we are doing just this. This handler is simply taking the JSON body that is sent into it, converting it to a list and then back into a JSON object and responding with the newly created JSON body.
Using curl to test this we can run and get the response the same JSON data returned.
curl -X POST morbo:8889 -d '{"number": 59,"somestring":"Hello, this is a test of the rest handler"}' -v
Hopefully this can help you call into an external REST web service or use CIC to handle inbound REST requests.
-Kevin